AngularJS、ReactJS、VueJSがあるこのご時世に今更BackboneJSかよ・・・。と思うかもしれませんが、まだまだシステムで使われているので勉強するのみなのです。
前提
概要
requireJSを使用してBackboneJSとその依存ファイル(jquery、underscore)を読み込み、Viewを作ってindex.htmlに「HelloWorld」を表示させる。
シンプルな設計です。
Viewについて何パターンかやります。
ディレクトリ構成
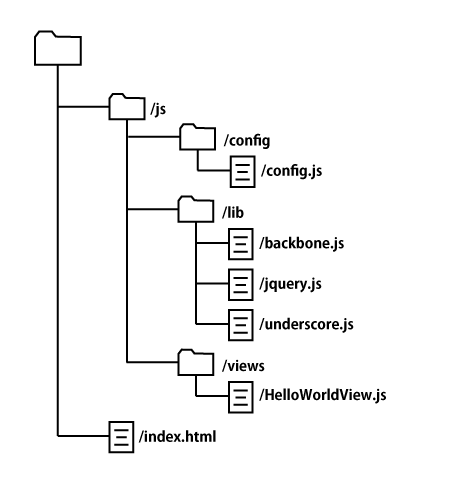
requireJSでbackboneJSを使えるようにする
index.html
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title></title>
</head>
<body>
<script src="https://requirejs.org/docs/release/2.3.6/minified/require.js"></script>
<script src="./js/config/config.js"></script>
<script src="./js/views/HelloWorldView.js"></script>
<h1>Hello World!</h1>
<div class="hoge"></div>
</body>
</html>
config.js
require.config({
baseUrl: "js",
paths: {
"backbone" : "lib/backbone",
"jquery" : "lib/jquery",
"underscore" : "lib/underscore"
},
shim: {
'backbone' : {
deps: ['underscore', 'jquery'],
exports: 'Backbone'
}
}
});
HelloWorldView.js
require(['backbone'], function(backbone) {
//index.html上のエレメント内に挿入する
var HelloWorldView = backbone.View.extend({
el: $('.hoge'),
})
var helloWorldView = new HelloWorldView();
$(helloWorldView.el).html('<h2>Hello world Backbone!!</h2>');
});
表示
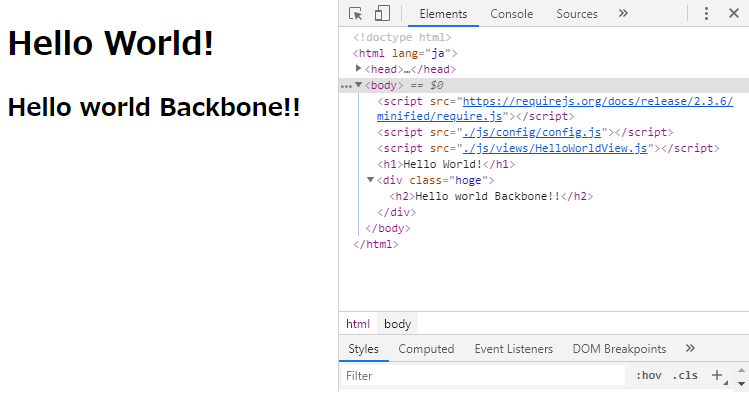
hogeという名前がついたdivタグにBackboneのViewから文字が挿入されたのが確認できます。
Viewで新たにElementを挿入する
index.html
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title></title>
</head>
<body>
<script src="https://requirejs.org/docs/release/2.3.6/minified/require.js"></script>
<script src="./js/config/config.js"></script>
<script src="./js/views/HelloWorldView.js"></script>
<h1>Hello World!</h1>
<div class="hoge"></div>
</body>
</html>
HelloWorld.js
require(['backbone'], function(backbone) {
//index.html上のエレメントに新たにタグを埋め込む
var HelloWorldView = backbone.View.extend({
tagName:"div",
className:"puge"
})
var helloWorldView = new HelloWorldView();
$('.hoge').append(helloWorldView.el);
$('.puge').html('<h2>Hello world Backbone!!</h2>');
});
表示
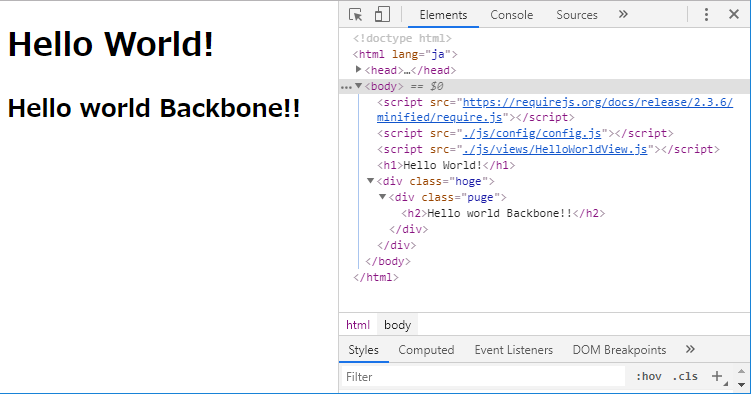
pugeという名前のdivタグごとhtmlに挿入されたのがわかります。
initializeを使用して描画処理を分割する
index.html
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title></title>
</head>
<body>
<script src="https://requirejs.org/docs/release/2.3.6/minified/require.js"></script>
<script src="./js/config/config.js"></script>
<script src="./js/views/HelloWorldView.js"></script>
<h1>Hello World!</h1>
<div class="hoge"></div>
</body>
</html>
HelloWorldView.js
require(['backbone'], function(backbone) {
//viewとして適切な分解をする
var HelloWorldView = backbone.View.extend({
el: $('.hoge'),
initialize: function() {
this.render();
},
render: function() {
$(this.el).html('<h2>Hello world Backbone!!</h2>');
}
});
new HelloWorldView();
});
表示
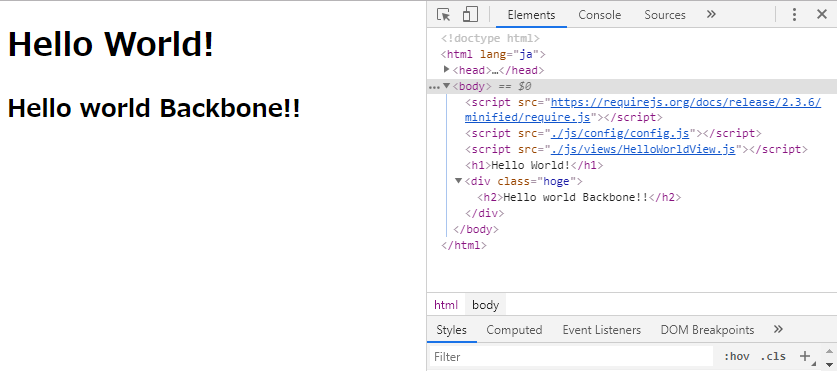
initialize関数はbackboneの初期処理を担当しています。
templateを使用してViewで描画する
underscore.jsにはtemplateが定義できる機能があり、viewでtemplateを呼び出して画面に描画してみます。
index.html
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title></title>
</head>
<body>
<script src="https://requirejs.org/docs/release/2.3.6/minified/require.js"></script>
<script src="./js/config/config.js"></script>
<script src="./js/views/HelloWorldView.js"></script>
<script type="text/template" id="hello-template">
<h2><%= text %></h2>
</script>
<h1>Hello World!</h1>
<div class="hoge"></div>
</body>
</html>
html上に外部定義としてtemplateを設定しています。
設定する時はtypeをtext/templateとし、templateを識別できるようにidを付けておきます。
「= text =」で囲まれた中にView側から文字を挿入します。
HelloWorld.js
require(['backbone'], function(backbone) {
//viewとして適切な分解をし、外部定義されたtemplateを呼び出す。
var HelloWorldView = backbone.View.extend({
el: $('.hoge'),
initialize: function() {
this.render();
},
render: function() {
var template = $('#hello-template').text();
var compiled = _.template(template);
var text = { "text": "Hello World Backbone!!"};
$(this.el).html(compiled(text));
}
});
new HelloWorldView();
});
表示
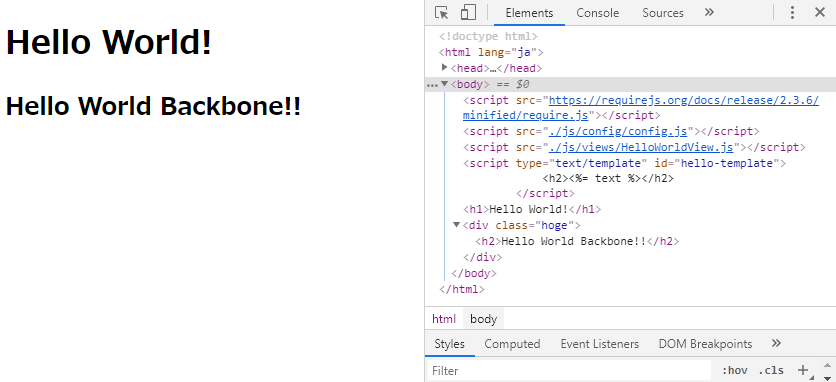
Viewで設定した文字がtemplateを通してhtml上に挿入されているのが分かります。
render関数で行ったtemplateの操作を1行で書くとこのようになります。
require(['backbone'], function(backbone) {
//viewとして適切な分解をする
var HelloWorldView = backbone.View.extend({
el: $('.hoge'),
initialize: function() {
this.render();
},
render: function() {
$(this.el).html(_.template($('#hello-template')
.text())({ "text": "Hello World Backbone!!"}));
}
});
new HelloWorldView();
});
まとめ
後半はbackbone.jsについての話になってしまいましたが、backbone.jsをrequireJSを使って読み込む時の方法でした。
コメントを書く